Arduino and Processing – Serial input
Serial output from Arduino to Processing. Reading a pulse modulation from potentiometer to processing.
First let’s set the Arduino circuit
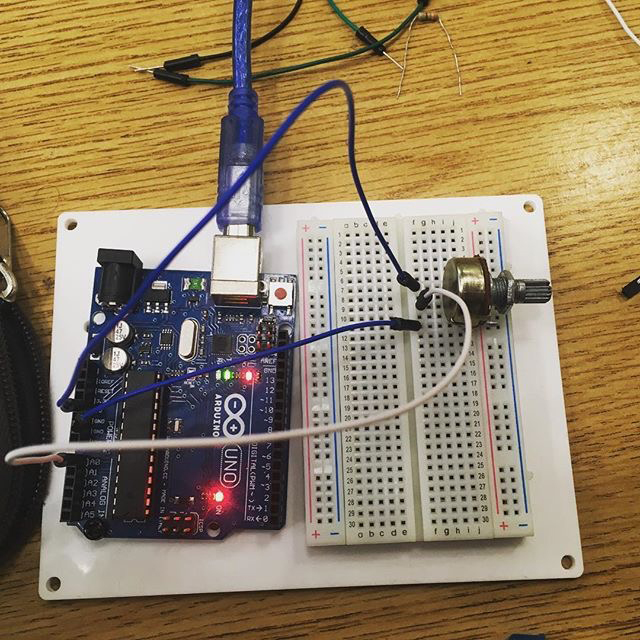
The Arduino code looks something like this. See below. Also, make sure your settings like the correct board and the port you are communicating with is the correct one.
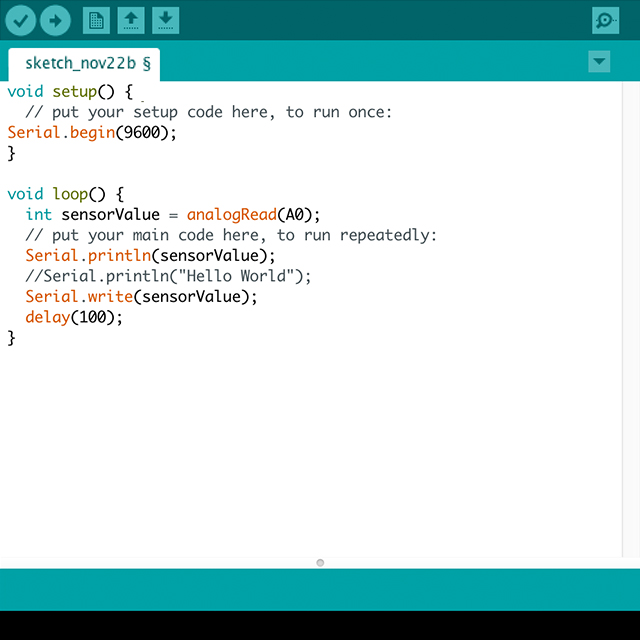
Now, let’s get the Processing code in. You’ll need to import the “Serial” library if you haven’t. See below.
import processing.serial.*;
int lf = 10; // Linefeed in ASCII
int value;
String myString = null;
Serial myPort; // The serial port
void setup() {
size(800,600);
background(0);
// List all the available serial ports
println(Serial.list());
// I know that the first port in the serial list on my mac
// is always my Keyspan adaptor, so I open Serial.list()[2].
// Open whatever port is the one you're using.
myPort = new Serial(this, Serial.list()[2], 9600);
myPort.clear();
// Throw out the first reading, in case we started reading
// in the middle of a string from the sender.
myString = myPort.readStringUntil(lf);
myString = null;
}
void draw() {
while (myPort.available() > 0) {
myString = myPort.readStringUntil(lf);
if (myString != null) {
myString = trim(myString);
value = int(myString);
println(value);
}
}
background(0);
rect(width/2, height, 100, -value/2);
}